Printing a shape with space Pattern using C
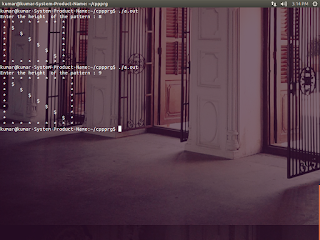
#include<stdio.h> void main() { int i,j,m; printf("Enter the height of the pattern : "); scanf("%d",&m); for(i=0;i<m;i++) { for(j=0;j<m;j++) { if(i==0 || i==m-1) printf(" * "); else if(j==0 || j==m-1) printf(" * "); else if(i==j) printf(" $ "); else printf(" "); } printf("\n"); } } o/p :